For this tutorial, we'll create a WCF RESTful application that will handle ONLY HTTP GET requests. The complete, and larger, HTTP POST - HTTP PUT & HTTP DELETE tutorial can be found HERE.
We'll start at an working MVC application, which will use an SQL database , showing as follows:
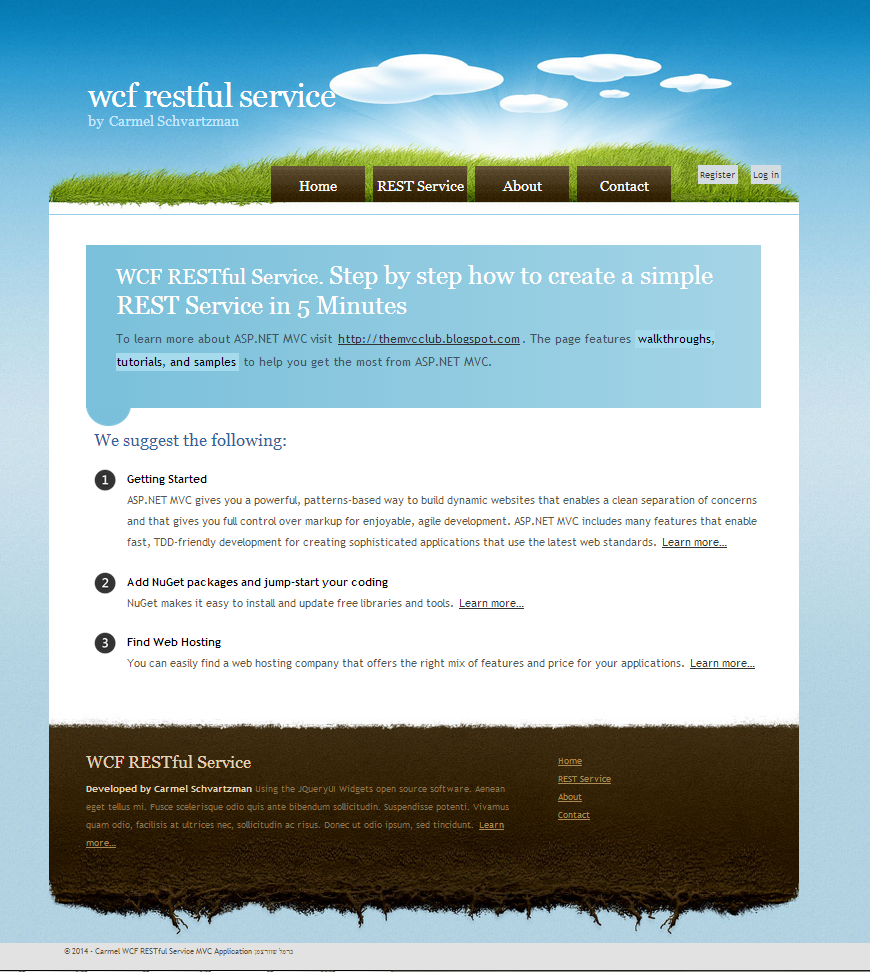
We'll then add a WCF Web Service to fetch data & render it in JSON format.
First check your app is running at the Visual Studio Development Server : that will allow you to check your WCF service bypassing possible security issues caused by using IIS:
Now add a new item to your project :
Search for all WCF items, and select AJAX-enabled WCF :
Take a look at the web.config automatically created: you'll recognize an ENDPOINT BEHAVIOUR which enables Web Script , and a webHttpBinding ENDPOINT linked to a CONTRACT named "BlogService" :
The "<enableWebScript>" directive allows the RESTful WCF service to be accessed through AJAX requests, and this option automatically includes the more basic "<WebHttp>" directive. This last option, WebHttp, is what transforms your WCF service in RESTful, that means, based on HTTP verbs to determine what CRUD operation is required (PUT = update, POST = create, GET = retrieve, DELETE = remove).
Setting "<enableWebScript>" is the same we did when creating ASMX Web Services, while setting the "[ScriptService]" attribute.
If you don't want your RESTful WCF service to handle AJAX requests, set just "WebHttp" in the Endpoint Behavior.
Therefore, open that "BlogService" class : it's tagged as a SERVICE CONTRACT , and inside there is a "DoWork" OPERATION :
Delete the function , and create your own OPERATION to fetch ALL the data :
Notice we serialize the data to JSON using the JavaScriptSerializer.
BUILD & RUN your service, and possibly you'll be faced with an exception:
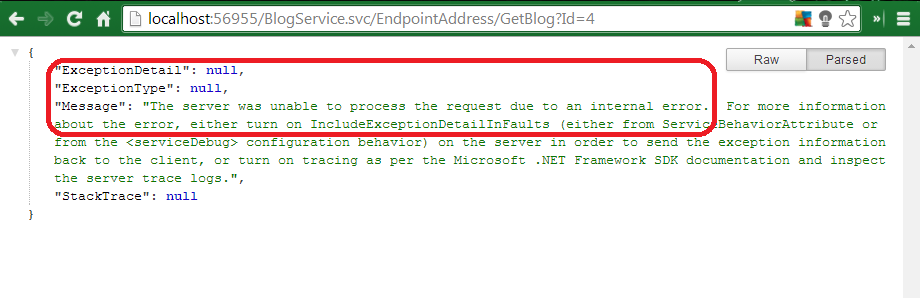
Put a breakpoint inside the OPERATION, and if you got the "CIRCULAR REFERENCE WAS DETECTED WHILE SERIALIZING AN OBJECT OF TYPE" :
...just add to the code "proxycreationenabled" = false, after the instantiation of the Entities Model :
The WCF Operation can return, instead of a string, all posts in a generic LIST<Blog> , as follows:
[ServiceContract(Namespace = "")]
public interface IBlogService
{
[OperationContract]
[WebGet(ResponseFormat = WebMessageFormat.Json)]
List<Blog> GetPosts();
[OperationContract]
[WebGet(ResponseFormat = WebMessageFormat.Json)]
Blog GetPost(string Id);
}
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class BlogService : IBlogService
{
BlogEntities ctx;
public BlogService()
{
ctx = new Models.BlogEntities();
ctx.Configuration.ProxyCreationEnabled = false;
}
public List<Blog> GetPosts()
{
List<Blog> data = ctx.Blogs.ToList();
return data;
}
public Blog GetPost(string Id)
{
int id = Convert.ToInt32(Id);
Blog data = ctx.Blogs.Where(b => b.BlogID == id).FirstOrDefault();
return data;
}
}
Getting the JSON results:
The same thing we can do to fetch ONLY ONE record, as a string :
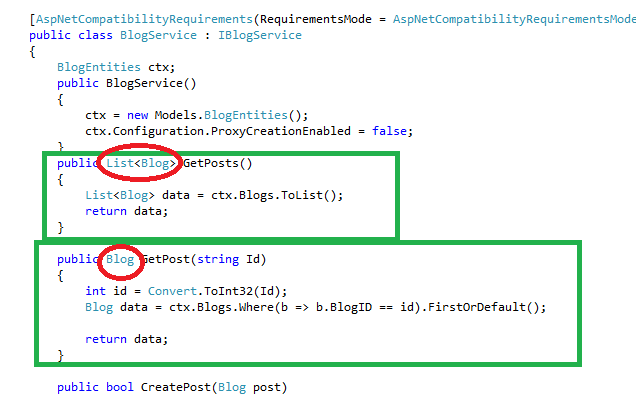
Rendering the JSON:
We're done. But, what's the URL address of the operation to get the data? Take a look at this screenshot to see from where comes the definition of every part of the URL path:
Happy programming.....
By Carmel Shvartzman
Therefore, open that "BlogService" class : it's tagged as a SERVICE CONTRACT , and inside there is a "DoWork" OPERATION :
Delete the function , and create your own OPERATION to fetch ALL the data :
Notice we serialize the data to JSON using the JavaScriptSerializer.
BUILD & RUN your service, and possibly you'll be faced with an exception:
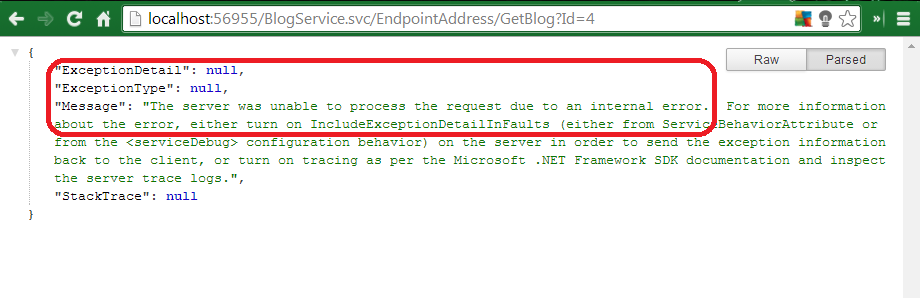
Put a breakpoint inside the OPERATION, and if you got the "CIRCULAR REFERENCE WAS DETECTED WHILE SERIALIZING AN OBJECT OF TYPE" :
...just add to the code "proxycreationenabled" = false, after the instantiation of the Entities Model :
The WCF Operation can return, instead of a string, all posts in a generic LIST<Blog> , as follows:
[ServiceContract(Namespace = "")]
public interface IBlogService
{
[OperationContract]
[WebGet(ResponseFormat = WebMessageFormat.Json)]
List<Blog> GetPosts();
[OperationContract]
[WebGet(ResponseFormat = WebMessageFormat.Json)]
Blog GetPost(string Id);
}
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class BlogService : IBlogService
{
BlogEntities ctx;
public BlogService()
{
ctx = new Models.BlogEntities();
ctx.Configuration.ProxyCreationEnabled = false;
}
public List<Blog> GetPosts()
{
List<Blog> data = ctx.Blogs.ToList();
return data;
}
public Blog GetPost(string Id)
{
int id = Convert.ToInt32(Id);
Blog data = ctx.Blogs.Where(b => b.BlogID == id).FirstOrDefault();
return data;
}
}
Getting the JSON results:
The same thing we can do to fetch ONLY ONE record, as a string :
[OperationContract]Or as a Blog object:
[WebGet]
public string GetBlogs()
{
Models.BlogEntities ctx = new Models.BlogEntities();
ctx.Configuration.ProxyCreationEnabled = false;
List<Blog> data = ctx.Blogs.ToList();
return new JavaScriptSerializer().Serialize(data);
}
[OperationContract]
[WebGet]
public string GetBlog(int Id)
{
Models.BlogEntities ctx = new Models.BlogEntities();
ctx.Configuration.ProxyCreationEnabled = false;
Blog data = ctx.Blogs.Where(b => b.BlogID == Id).FirstOrDefault();
return new JavaScriptSerializer().Serialize(data);
}
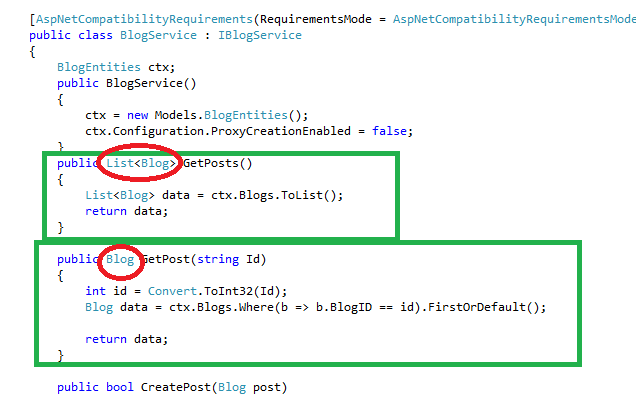
Rendering the JSON:
We're done. But, what's the URL address of the operation to get the data? Take a look at this screenshot to see from where comes the definition of every part of the URL path:
That's all!!
In this tutorial we've learn how to create a very simple Ajax enabled WCF RESTful application in Asp.Net MVC in 5 minutes. Happy programming.....
By Carmel Shvartzman
כתב: כרמל שוורצמן
No comments:
Post a Comment